When we create an account on a website we provide our details and also we create a password to access that account later on. All our details go to the organization’s database including password. So if the password is stored in plain text, and the database gets compromised there is a very good chance that our account can be accessed by intruders using our password.
Encrypting a password and then storing it in a database can solve this problem. Encryption ensures that the password is stored in such a way that an intruder is unable to decrypt it.
We have a module called “bcrypt” that we can install and use in our NodeJs application. Bcrypt uses a hashing algorithm to encrypt passwords before storing it in the database.
So let us see how can we install this package module and use it in our NodeJs application to encrypt the password.
STEP 1: Install and configure the bcrypt npm in your application directory
npm install bcryptOnce the dependencies are installed we need to require bcrypt in our application and declare a variable known as salt.
STEP 2: Require bcrypt in Nodejs file and declare ‘salt’.
Const bcrypt = require(‘bcrypt’) ;Var salt = 10 ;
Here salt variable is nothing but a cost factor that determines how much time is required to create a bcrypt hash string. We generally give a random number to this variable. The greater the number we choose, the number of hashing rounds are done and therefore it will be highly secured. The value can be changed for different calculations and this will, therefore, provide different results even if the same password is entered by two different users.
STEP 3: Create an account and generate a bcrypt hash.
Create a route in your application for signup.
Now we need to pass the password, that the user has created, a salt variable that we declared and a callback function. This callback function will return an error and encrypted string.
bcrypt.hash() function will auto-generate an encrypted hash string and now you can insert all the data entered by the user along with the password in the database. The password entered by the user would be an encrypted string.
bcrypt.hash(req.body.values.password, salt, (err, encrypted) => {Req.body.values.password = encrypted ;
services.signup(req.body, otp).then((userCreated) => {
response.status(200).send(userCreated);
})
})
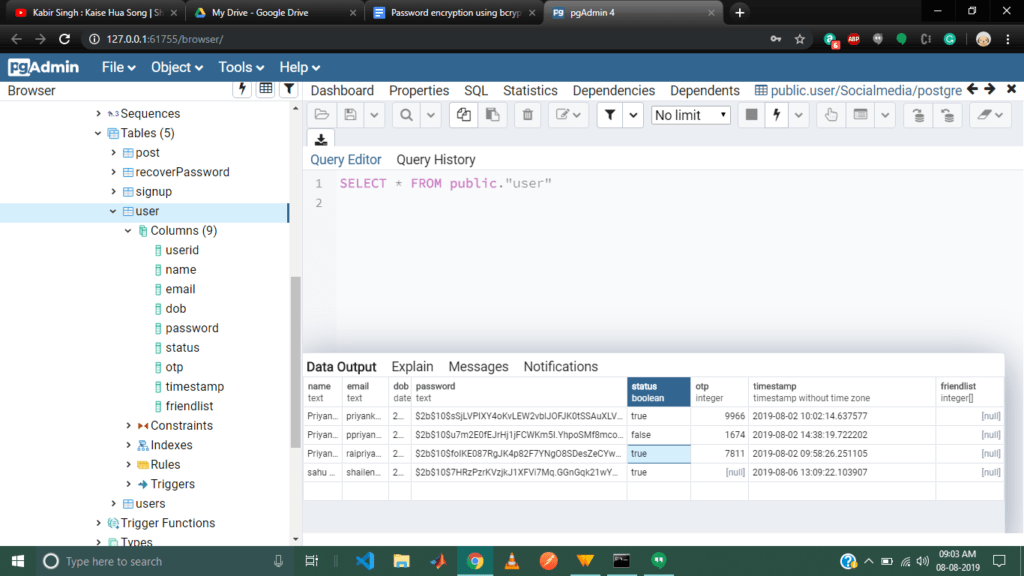
Encrypted password stored in the database
STEP 4: Compare the encrypted string with the password at the time of login.
Create a route for login, this will verify if the user has already registered and has entered the correct password. Now check if the email, that the user has entered is present in the database or not. If it is present then check if email and passwords match with those stored in the database. Either you can do by yourself or you can hire nodejs developers & I suggest you hire reactjs developers for fron-end development.
For this we use brcypt.compare() function. This function will take the password entered by the user at the time of login and the encrypted password from the database and matches it. If both the password matches, login is successful and the user is directed to the homepage.
bcrypt.compare(req.params.password,password,function(err,result) {
if (result == true){
services.login(req.params.name,password).then((userFound)=>
{
response.send(“Password is Correct”);
})
} else {
response.send(“Password is Incorrect”);
}
})
Using bcrypt for password encryption is very easy and useful for people working with Nodejs. It is considered to be the best practice to store the password as a hashed string rather than plain text to provide security to the user’s data.
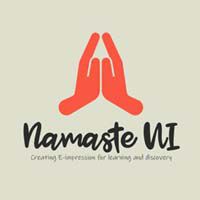
Namaste UI collaborates closely with clients to develop tailored guest posting strategies that align with their unique goals and target audiences. Their commitment to delivering high-quality, niche-specific content ensures that each guest post not only meets but exceeds the expectations of both clients and the hosting platforms. Connect with us on social media for the latest updates on guest posting trends, outreach strategies, and digital marketing tips. For any types of guest posting services, contact us on info[at]namasteui.com.