What is Attribute Routing in MVC?
Attribute Routing use attributes to define routes as a controller level, action level.
In fact, it gives more flexibility over URL.Attribute routing enables .NET development companies to simplify their routing processes, especially when they have a need for enterprise application development.
Route Prefix in Attribute Routing
Route Prefixes are used to prefix for any route apply to the action method. It is used to set a common prefix for a controller that you want to apply in the action method. we need to define the route prefix on the controller so the action method use prefix.
Let us understand practically how attribute routing works using Asp.Net MVC.
Here, I am creating a model for the controller class.
namespace AJAX.Models
{
public class User
{
public int U_Id{ get; set; }
public string U_Name{ get; set; }
}
}
I am creating UsersController class and add the route attribute in the action method.
namespace AJAX.Controllers { public class UsersController : Controller { static List<User> users = new List<User>() { new User() { U_Id = 1, U_Name= "Vaffeljer" }, new User() { U_Id = 2, U_Name= "AlfredsHorn" }, new User() { U_Id = 3, U_Name= "BerglundsHerodas" }, new User() { U_Id = 4, U_Name= "Sammbit" }, new User() { U_Id = 5, U_Name= "Pranayi" }, new User() { U_Id = 6, U_Name= "GodasCarnes" } }; [HttpGet] [Route("User")] public ActionResultGetAllUsers() { return View(users); } [HttpGet] [Route("User/{userID}")] public ActionResultGetUsertByID(int userID) { User userDetails = users.FirstOrDefault(s =>s.U_Id == userID); return View(userDetails); } [HttpGet] [Route("User/{userID}/courses")] public ActionResultGetUserCourses(int userID) { List<string>CourseList = new List<string>(); if (userID == 1) CourseList = new List<string>() { "ASP.NET", "VB.NET", "SQL Server" ,"Web Api"}; else if (userID == 2) CourseList = new List<string>() { "ASP.NET MVC", "C#.NET", "ADO.NET" ,"MySQL" }; else if (userID == 3) CourseList = new List<string>() { "ASP.NET WEB API", "C#.NET", "Entity Framework", "Web Api" }; else if (userID == 4) CourseList = new List<string>() { "ASP.NET WEB API", "VB.NET", "Entity Framework" }; else if (userID == 5) CourseList = new List<string>() { "ASP.NET WEB API", "F#.NET", "Entity Framework" ,""}; else CourseList = new List<string>() { "Bootstrap", "jQuery", "AngularJs" ,"ReactJs" ,"AJAX" }; ViewBag.CourseList = CourseList; return View(); } } }
In this example, I am using the route attribute at the action level to define the route. Instead of Users, I define a route as a User and I want all the action methods to start with the same prefix I.e. that means User is the common prefix for all the routes in Users Controller class.
Instead of defining prefix at each action method, we can use route prefix to define a prefix for all action methods. In this example, the common prefix User is specified for the entire Users Controller using the [RoutePrefix] attribute at the controller level
namespace AJAX.Controllers { [RoutePrefix("User")] public class UsersController : Controller { //private countryDbContextdb = new countryDbContext(); // GET: Users static List<User> users = new List<User>() { new User() { U_Id = 1, U_Name= "Vaffeljer" }, new User() { U_Id = 2, U_Name= "AlfredsHorn" }, new User() { U_Id = 3, U_Name= "BerglundsHerodas" }, new User() { U_Id = 4, U_Name= "Sammbit" }, new User() { U_Id = 5, U_Name= "Pranayi" }, new User() { U_Id = 6, U_Name= "GodasCarnes" } }; [HttpGet] [Route] public ActionResultGetAllUsers() { return View(users); } [HttpGet] [Route("{userID}")] public ActionResultGetUsertByID(int userID) { User userDetails = users.FirstOrDefault(s =>s.U_Id== userID); return View(userDetails); } [HttpGet] [Route("{userID}/courses")] public ActionResultGetUserCourses(int userID) { List<string>CourseList = new List<string>(); if (userID == 1) CourseList = new List<string>() { "ASP.NET", "VB.NET", "SQL Server" ,"Web Api"}; else if (userID == 2) CourseList = new List<string>() { "ASP.NET MVC", "C#.NET", "ADO.NET" ,"MySQL" }; else if (userID == 3) CourseList = new List<string>() { "ASP.NET WEB API", "C#.NET", "Entity Framework", "Web Api" }; else if (userID == 4) CourseList = new List<string>() { "ASP.NET WEB API", "VB.NET", "Entity Framework" }; else if (userID == 5) CourseList = new List<string>() { "ASP.NET WEB API", "F#.NET", "Entity Framework" ,""}; else CourseList = new List<string>() { "Bootstrap", "jQuery", "AngularJs" ,"ReactJs" ,"AJAX" }; ViewBag.CourseList = CourseList; return View(); } } }
With the help of the Route Prefix attribute, we can eliminate the need to repeat the common prefix at each action method in the controller class we just define the common prefix at the controller level using the route prefix attribute.

[Fig: – RoutePrefix]
In this image, you can show that instead of Users in URL it prints User in URL.
Override Route Prefix in Attribute Routing
When we need to override the route attribute need to use the (~) sign with the route attribute it overrides the RoutePrefix.
namespace AJAX.Controllers { [RoutePrefix("User")] public class UsersController : Controller { [HttpGet] [Route("UserCourse/{userID}")] public ActionResultGetUserCourses(int userID) { List<string>CourseList = new List<string>(); if (userID == 1) CourseList = new List<string>() { "ASP.NET", "VB.NET", "SQL Server" ,"Web Api"}; else if (userID == 2) CourseList = new List<string>() { "ASP.NET MVC", "C#.NET", "ADO.NET" ,"MySQL" }; else if (userID == 3) CourseList = new List<string>() { "ASP.NET WEB API", "C#.NET", "Entity Framework", "Web Api" }; else if (userID == 4) CourseList = new List<string>() { "ASP.NET WEB API", "VB.NET", "Entity Framework" }; else if (userID == 5) CourseList = new List<string>() { "ASP.NET WEB API", "F#.NET", "Entity Framework" ,""}; else CourseList = new List<string>() { "Bootstrap", "jQuery", "AngularJs" ,"ReactJs" ,"AJAX" }; ViewBag.CourseList = CourseList; return View(); } } }
In this example, I want to use the UserCourseprefix for the GetUserCourses action method instead of RoutePrefix value.
If we use the Route attribute on the GetUserCourses action method as shown above and when we navigate to UserCourse/1 we get the resource not found error.

[Fig:- Resource not found]
When we navigate User/UserCourse/1 it will give output. In this URL we need to eliminate the route prefix User instead of User it displays UserCourse.
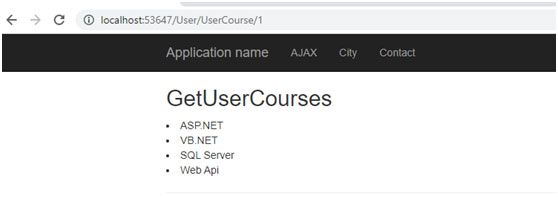
[Fig:- Route prefix override]
So we need to override the route prefix using the ( ~ )sign.
[HttpGet] [Route("~/UserCourse/{userID}")] public ActionResultGetUserCourses(int userID) { List<string>CourseList = new List<string>(); if (userID == 1) CourseList = new List<string>() { "ASP.NET", "VB.NET", "SQL Server" ,"Web Api"}; else if (userID == 2) CourseList = new List<string>() { "ASP.NET MVC", "C#.NET", "ADO.NET" ,"MySQL" }; else if (userID == 3) CourseList = new List<string>() { "ASP.NET WEB API", "C#.NET", "Entity Framework", "Web Api" }; else if (userID == 4) CourseList = new List<string>() { "ASP.NET WEB API", "VB.NET", "Entity Framework" }; else if (userID == 5) CourseList = new List<string>() { "ASP.NET WEB API", "F#.NET", "Entity Framework" ,""}; else CourseList = new List<string>() { "Bootstrap", "jQuery", "AngularJs" ,"ReactJs" ,"AJAX" }; ViewBag.CourseList = CourseList; return View(); }

[Fig:- Override RoutePrefix]
After running this code, we navigate to URL UserCourse/1.
Conclusion
Route Prefix in attribute routing allows us to specify a common prefix for all action method of controller class instead of defining prefix at every action method route prefix also allow us to override the route prefix value.
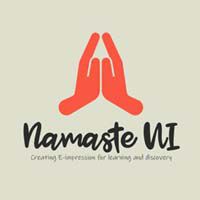
Namaste UI collaborates closely with clients to develop tailored guest posting strategies that align with their unique goals and target audiences. Their commitment to delivering high-quality, niche-specific content ensures that each guest post not only meets but exceeds the expectations of both clients and the hosting platforms. Connect with us on social media for the latest updates on guest posting trends, outreach strategies, and digital marketing tips. For any types of guest posting services, contact us on info[at]namasteui.com.
Useful Information. Thanks for sharing.